| 

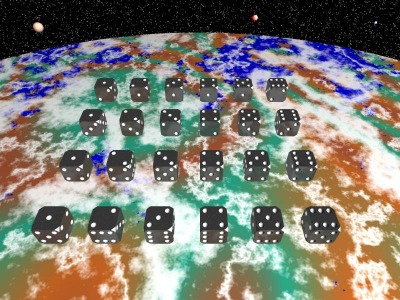
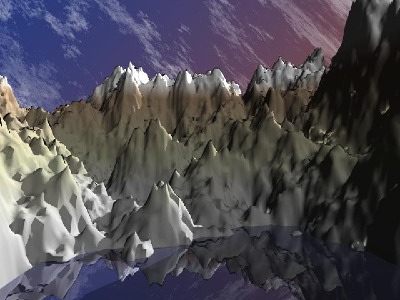
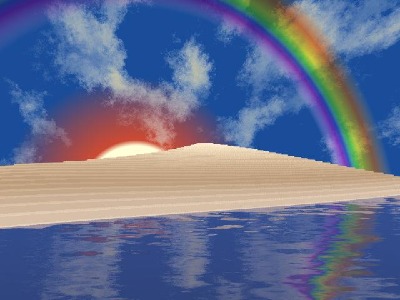



zunächst einige Zwischenstufen des Algorithmus...
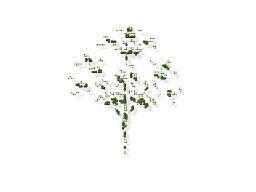
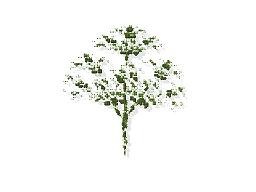
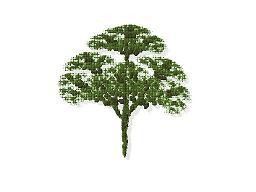
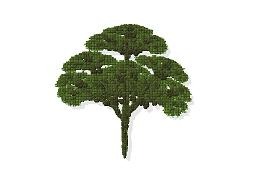
und hier die Ergebisse...
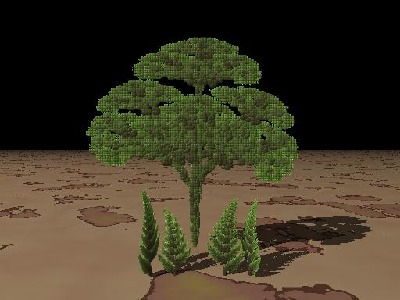
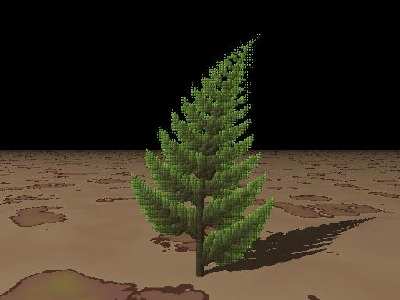
Für Interessierte gibt es hier den Quelltext des Makros, die Benutzung ist im Header erklärt. Weiter unten sind einige der Textdateien zum Erzeugen spezieller Pflanzen, die durch das Makro miteingebunden werden.

/****************************************************************************************** * ------------------------------------------- * IFSPlants * * macro for creating 2-dimensional plants * ------------------------------------------- * * by Marcus Lauks, 06/2005 * * * usage of the macro: * * - include "IFSPlants.inc" in your .pov file * - start macro with the according text file which contains * the parameters for each special object * example: #declare tree = object { IFSPlant("MyPlant.txt") } * - use object * example: object { tree scale 2 translate <-100,0,100>} * * usage of the text file: * - name of parameter (number of parameters): description * * - dimension (1): size of object [dimesion][dimension] * - depth (1): number of iterations, results in increasing density * - dontShow (1): don't show first pics because they don't match * - dotSize (1): initial dot size * - dotAmbient (1): dot ambience * - dotPhong (1): dot phong * - N (1): number of functions per iteration step * - range (4): xmin, xmax, ymin, ymax (affects the appearance of the object) * - ko[N][0..5] (N*6): array of six coefficients for each of N functions * - (for matrix operations rotation, scaling and displacement) * - start object (reads till EOF): pairs of x-/ y- coordinates for the start object * (doesn'n really affect the final result, must be at least one point) * * ToDo: * * - parameter "dimension" is set to "#declare" to enable usage in .pov file, * for restriction to the scope of the macro change to "#local" * *******************************************************************************************/
#macro IFSPlant(textFile)
#include "arrays.inc"
#fopen dataFile textFile read
/************************/ /* text file parameters */ /************************/ #declare dimension=0; #local depth=0; #local dontShow=0; #local dotSize=0.0; #local dotAmbient=0.0; #local dotPhong=0.0; #local N=0;
/****************************/ /* read data from text file */ /****************************/
// read parameters #read (dataFile, dimension) #read (dataFile, depth) #read (dataFile, dontShow) #read (dataFile, dotSize) #read (dataFile, dotAmbient) #read (dataFile, dotPhong) #read (dataFile, N)
// init coefficients #local ko = array[N][6] { { 0.0, 0.0, 0.0, 0.0, 0.0, 0.0 } { 0.0, 0.0, 0.0, 0.0, 0.0, 0.0 } { 0.0, 0.0, 0.0, 0.0, 0.0, 0.0 } { 0.0, 0.0, 0.0, 0.0, 0.0, 0.0 } }
// init graph1, graph2 #local graph1 = array[dimension][dimension]; #local graph2 = array[dimension][dimension]; #local i=0; #local j=0; #while (j<dimension) #local i=0; #while (i<dimension) #local graph1[i][j]=0; #local graph2[i][j]=0; #local i=i+1; #end #local j=j+1; #end
// read range (min, max) #local xrmin=0; #local xrmax=0; #local yrmin=0; #local yrmax=0; #local xfac=0; #local yfac=0; #read (dataFile, xrmin, xrmax, yrmin, yrmax) #local xfac=dimension/(xrmax-xrmin); #local yfac=dimension/(yrmax-yrmin);
// read coefficients #local a=0; #local b=0; #while (a<N) #local b=0; #while (b<6) #read (dataFile, ko[a][b]) #local b=b+1; #end #local a=a+1; #end
// read start values graph1 #local _x=0; #local _y=0; #while (defined(dataFile)) #read (dataFile, _x, _y) #local graph1[dimension/2+_x][_y]=1; #end
/******************/ /* color settings */ /******************/
#local Rb=50/255; // start values for color morph function (dark brown) #local Gb=40/255; #local Bb=30/255; #local Rg=140/255; // end values for color morph function (light green) #local Gg=220/255; #local Bg=70/255;
/////////////////////////////////////////////////////////////////////////////
/***********************/ /* calculate the plant */ /***********************/
#local X=0; #local Y=0; #local V=0; #local W=0; #local R=1; #local G=1; #local B=1; #local ds=dotSize; #local xneu=0; #local yneu=0; #local c=1;
union { /************/ /* IFS-LOOP */ /************/ #while (c<=depth) /************************************/ /* color morph function */ /* change color from brown to green */ /************************************/ // linear //#local R=Rb-(Rb-Rg)*(c-1)/depth; //#local G=Gb-(Gb-Gg)*(c-1)/depth; //#local B=Bb-(Bb-Bg)*(c-1)/depth; // exponential #local R=Rb-(Rb-Rg)*(1-exp((c-1)/depth))/(1-exp(1)); #local B=Bb-(Bb-Bg)*(1-exp((c-1)/depth))/(1-exp(1)); #local G=Gb-(Gb-Gg)*(1-exp((c-1)/depth))/(1-exp(1)); //change dotSize from 100% (c=1) to about 0% (c=depth) #local ds=dotSize*(1-(c-1)/depth); /********************/ /* calculate graph2 */ /********************/ #local i=0; #local j=0; #while (j<dimension) #local i=0; #while (i<dimension) #if (graph1[i][j]=1) #local X=i/xfac+xrmin; #local Y=j/yfac+yrmin; #local n=0; #while (n<N) #local V=ko[n][0]*X+ko[n][1]*Y+ko[n][2]; #local W=ko[n][3]*X+ko[n][4]*Y+ko[n][5]; #local xneu=(V-xrmin)*xfac; #local yneu=(W-yrmin)*yfac; #if (xneu<dimension & xneu>=0 & yneu<dimension & yneu>=0) #local graph2[xneu][yneu]=1; #end #local n=n+1; #end #end #local i=i+1; #end #local j=j+1; #end /*************************/ /* copy graph2 to graph1 */ /*************************/ #local i=0; #local j=0; #while (j<dimension) #local i=0; #while (i<dimension) #local graph1[i][j]=graph2[i][j]; #local i=i+1; #end #local j=j+1; #end /************************/ /* add graph2 to object */ /************************/ #local i=0; #local j=0; #while (j<dimension) #local i=0; #while (i<dimension) #if (graph2[i][j]=1 & c>dontShow) //don't show first pics sphere { <i-dimension/2,j,0>, ds //translate to x=0 pigment {color rgb<R,G,B>} finish {ambient dotAmbient phong dotPhong} } #end #local i=i+1; #end #local j=j+1; #end /***************/ /* init graph2 */ /***************/ #local i=0; #local j=0; #while (j<dimension) #local i=0; #while (i<dimension) #local graph2[i][j]=0; #local i=i+1; #end #local j=j+1; #end #local c=c+1; #end // end of IFS-LOOP } // end of union
#end // end of macro

100, 12, 2, 1.5, 0.6, 0.2, 4, -3, 3, -3, 4,
-0.04, 0, -0.08, -0.23, -0.65, 0.26,
0.61, 0, 0.07, 0, 0.31, 2.5,
0.65, 0.29, 0.54, -0.3, 0.48, 0.39,
0.64, -0.3, -0.56, 0.16, 0.56, 0.4,
1, 2, 2, 1, 3, 0, 4, 1, 5, 2, 6, 3, 0, 3, 1, 4, 2, 5, 3, 6, 4, 5, 5, 4,

100, 20, 2, 1.8, 0.6, 0.2, 4,
-4, 4, 0, 10, 0, 0, 0, 0, 0.16, 0,
0.85, 0.04, 0, -0.04, 0.85, 1.6,
0.2, -0.26, 0, 0.23, 0.22, 1.6,
0.15, 0.28, 0, 0.26, 0.24, 0.44,
1, 2, 2, 1, 3, 0, 4, 1, 5, 2, 6, 3, 0, 3, 1, 4, 2, 5, 3, 6, 4, 5, 5, 4,

100, 20, 2, 1.8, 0.6, 0.2, 4,
-4, 4, 0, 10, 0, 0, 0, 0, 0.26, 0,
0.85, 0.04, 0, -0.04, 0.85, 1.6,
0.2, -0.26, 0, 0.23, 0.22, 1.6,
0.15, 0.8, 0, 0.26, 0.24, 0.44,
1, 2, 2, 1, 3, 0, 4, 1, 5, 2, 6, 3, 0, 3, 1, 4, 2, 5, 3, 6, 4, 5, 5, 4,

100, 17, 1, 1.8, 0.6, 0.2, 4,
-6, 6, 0, 12, 0, 0, 0, 0, 0.16, 0,
0.85, 0.04, 0, -0.04, 0.85, 1.6,
0.2, -0.26, 0, 0.23, 0.22, 1.6,
-0.15, 0.28, 0, 0.26, 0.24, 0.44,
1, 2, 2, 1, 3, 0, 4, 1, 5, 2, 6, 3, 0, 3, 1, 4, 2, 5, 3, 6, 4, 5, 5, 4,
|
|